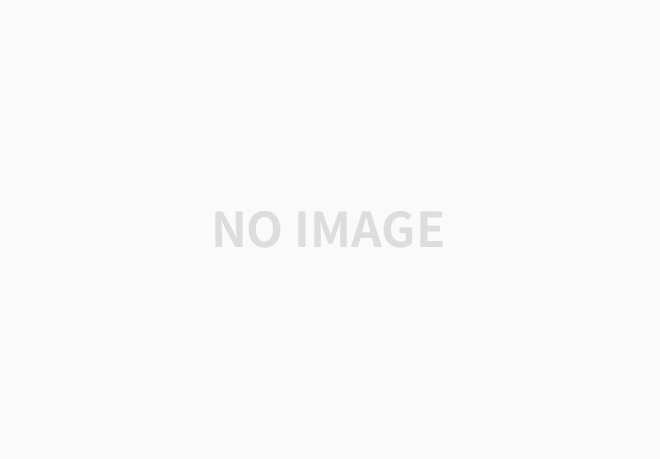
Process 와 Thread 간의 관계를 이해하기 쉽게 프로세스를 다시 정의하자면, 프로세스는 Task 와 Thread 를 더한 것이다. 다시 말해 프로세스가 처리해야 하는 어떠한 작업, 그리고 그 작업을 수행하는 작업자를 합쳐서 프로세스라 부르는 것이다. 궁극적으로 쓰레드들이 특정 작업을 처리하게 되므로 쓰레드는 CPU이용의 기본 단위가 된다. 전통적인 Heavyweight 프로세스는 하나의 Thread of Control 을 (제어 쓰레드) 갖고 있다. 만일 다수의 제어 쓰레드가 있다면 이 프로세스는 동시에 여러개의 작업을 처리할 수 있다. 단일 쓰레드 프로세스와 다중 쓰레드 프로세스의 차이점을 위의 그림에서 확인하자.
Thread 의 구성 요소
* Thread ID
* Program Counter
* Register
* Stack
* 다른 Thread 들과 공유하는 코드영역, 데이터 영역, 열린 파일이나 시그널 같은 운영체제의 자원들.
#include <pthread.h>
#include <stdio.h>
int sum; //shared by threads
void *runner(void *param); //the thread
int main(int argc, char *argv[]){
pthread_t tid; //the thread identifier
pthread_attr_t attr; //set of attributes for the thread
if(argc != 2){
fprintf(stderr, "usage :a.out <integer value>\n");
return -1;
}
if(atoi(argv[1]) < 0){
fprintf(stderr, "%d must be >= 0\n", atoi(argv[1]));
return -1;
}
//get the default attributes
pthread_attr_init(&attr);
//create the thread
pthread_create(&tid, &attr, runner, argv[1]);
//wait for the thread to exit
pthread_join(tid, NULL);
printf("sum = %d\n", sum);
}
void *runner(void *param){
int i, upper = atoi(param);
sum = 0;
for(i = 1; i <= upper; i++){
sum += i;
}
pthread_exit(0);
}